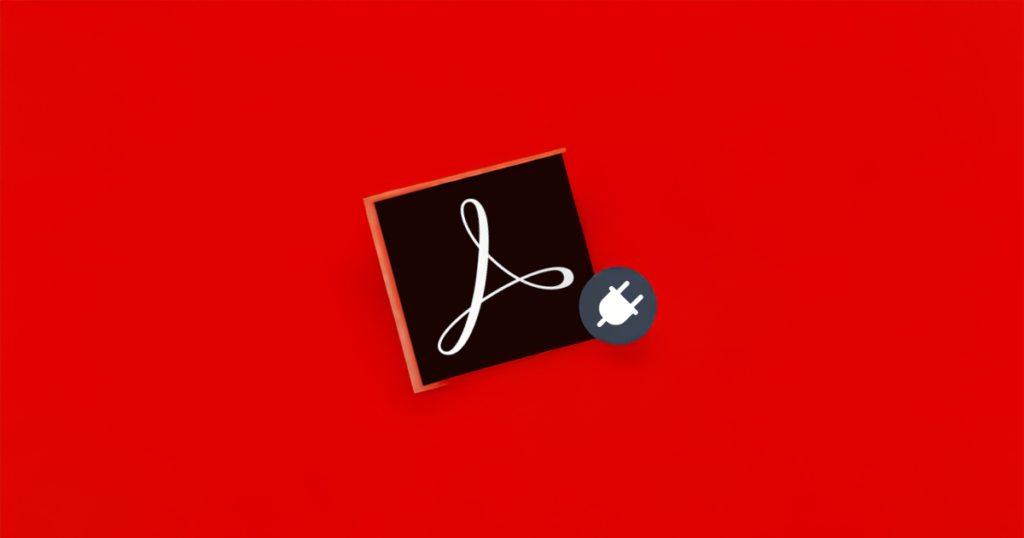
Introduction
Adobe Acrobat is a versatile tool for handling PDF documents, but sometimes you might need functionality that’s not available out of the box. Creating custom plug-ins allows you to extend Acrobat’s capabilities to suit your specific needs. In this guide, we’ll show you how to develop an Adobe Acrobat plug-in that adds a custom menu item to display a “Hello, World!” alert.
Table of Contents
- Understanding Adobe Acrobat Plug-ins
- Setting Up Your Development Environment
- Creating Your First Plug-in with a Custom Menu Item
- Exploring the Acrobat SDK
- Best Practices for Plug-in Development
- Deploying Your Plug-in
- Conclusion
Understanding Adobe Acrobat Plug-ins
What Are Plug-ins?
Plug-ins are software components that add specific features to an existing application. In the context of Adobe Acrobat, plug-ins allow developers to enhance or modify the application’s functionality.
Why Create a Plug-in?
- Customization: Tailor Acrobat to meet specific workflow requirements.
- Automation: Automate repetitive tasks to improve efficiency.
- Integration: Connect Acrobat with other software systems.
Setting Up Your Development Environment
Before you start coding, you need to set up your development environment.
Prerequisites
- Programming Knowledge: Proficiency in C or C++.
- Development Tools: An Integrated Development Environment (IDE) like Microsoft Visual Studio (for Windows) or Xcode (for macOS).
- Adobe Acrobat SDK: The Software Development Kit provided by Adobe.
Downloading the Adobe Acrobat SDK
- Visit the Adobe Developer Website: Go to the Adobe Acrobat Developer Center.
- Download the SDK: Navigate to the SDK section and download the latest version compatible with your system.
Installing the SDK
Extract the downloaded SDK to a convenient location on your hard drive. The SDK includes:
- Header Files: For API functions.
- Sample Code: Examples to help you get started.
- Documentation: Essential reading to understand the SDK’s capabilities.
By default, the SDK is placed into the Program Files/Adobe folder on Windows systems. However, if you intend to compile and/or make modifications to the various samples included with the SDK, you will likely want to relocate the SDK to a different directory. This is recommended particularly due to potential user rights restrictions that often arise when trying to access or manipulate files in the Program Files directory. Moving the SDK to a more accessible location can streamline the process and alleviate any permissions issues that might impede your development efforts, allowing for a more efficient workflow.
Setting Up Your IDE
For Windows (Visual Studio)
- Create a New Project: Choose a Win32 Project and set it as a DLL.
- Include Directories: Add the SDK’s
Include
andSource
directories to your project’s include path. - Library Directories: Link against the necessary Adobe Acrobat libraries found in the SDK.
- Preprocessor Definitions: Add
WIN_PLATFORM
,ACRO_SDK_PLUGIN
, andWIN_ENV
to your preprocessor definitions.
For macOS (Xcode)
- Create a New Project: Select a Dynamic Library project.
- Include Directories: Add the SDK’s
Include
andSource
directories. - Frameworks: Link the required frameworks provided by the SDK.
- Preprocessor Definitions: Add
MAC_PLATFORM
,ACRO_SDK_PLUGIN
, andMAC_ENV
to your preprocessor definitions.
Creating Your First Plug-in with a Custom Menu Item
Let’s build a plug-in that adds a custom menu item to Acrobat’s menu bar. When the menu item is clicked, it will display a “Hello, World!” alert.
Step 1: Define the Plug-in Handshake
The handshake functions initialize your plug-in and allow Acrobat to recognize it.
#include "PIHeaders.h"
ACCB1 ASBool ACCB2 PluginExportHFTs(void) {
return true;
}
ACCB1 ASBool ACCB2 PluginImportReplaceAndRegister(void) {
return true;
}
ACCB1 ASBool ACCB2 PluginInit(void);
ACCB1 void ACCB2 PluginUnload(void);
ASAtom GetExtensionName(void);
Step 2: Implement the Plug-in Initialization and Unload Functions
ACCB1 ASBool ACCB2 PluginInit(void) {
// Call the function to add the menu item
AddOurMenuItem();
return true;
}
ACCB1 void ACCB2 PluginUnload(void) {
// Cleanup code here (if necessary)
}
ASAtom GetExtensionName(void) {
return ASAtomFromString("HelloWorldPlugin");
}
Step 3: Add the Custom Menu Item
We need to define a function that adds a menu item to Acrobat’s UI.
// Function Prototypes
void AddOurMenuItem(void);
ACCB1 void ACCB2 MyMenuItemCallback(void *clientData);
// Function Implementations
void AddOurMenuItem(void) {
// Get the menu bar
AVMenuBar menuBar = AVAppGetMenubar();
// Create a new menu item
AVMenuItem menuItem = AVMenuItemNew("Say Hello", "SayHelloMenuItem", NULL, false, NO_SHORTCUT, 0, NULL);
// Create or get a submenu (e.g., under the "Plug-Ins" menu)
AVMenu pluginsMenu = AVMenubarAcquireMenuByName(menuBar, "ADBE:PlugIns");
if (pluginsMenu == NULL) {
// If the "Plug-Ins" menu doesn't exist, create it
pluginsMenu = AVMenuNew("Plug-Ins", "ADBE:PlugIns", NULL, false);
AVMenubarAddMenu(menuBar, pluginsMenu, APPEND_MENU);
}
// Add the menu item to the submenu
AVMenuAddMenuItem(pluginsMenu, menuItem, APPEND_MENUITEM);
// Release the menu
AVMenuRelease(pluginsMenu);
// Set the callback for the menu item
AVMenuItemSetExecuteProc(menuItem, ASCallbackCreateProto(AVExecuteProc, MyMenuItemCallback), NULL);
// Release the menu item
AVMenuItemRelease(menuItem);
}
ACCB1 void ACCB2 MyMenuItemCallback(void *clientData) {
AVAlertNote("Hello, World!");
}
Step 4: Compile the Plug-in
- Build Configuration: Set your build configuration to match Acrobat’s architecture (32-bit or 64-bit).
- Include Paths: Ensure that the SDK’s
Include
andSource
directories are included. - Library Paths: Link against the necessary libraries from the SDK.
- Compile: Build the project to generate the plug-in file (
.api
on Windows,.acroplugin
on macOS).
Step 5: Install the Plug-in
Copy the compiled plug-in to Acrobat’s plug-ins directory:
- Windows:
C:\Program Files\Adobe\Acrobat DC\Acrobat\plug_ins
- macOS:
/Applications/Adobe Acrobat DC/Adobe Acrobat.app/Contents/Plug-ins
Step 6: Test the Plug-in
- Launch Acrobat: Open Adobe Acrobat.
- Verify the Menu Item: Look for your new menu item under the “Plug-Ins” menu.
- Test the Functionality: Click on the “Say Hello” menu item. The “Hello, World!” alert should appear.
Exploring the Acrobat SDK
To build more complex plug-ins, familiarize yourself with the SDK’s features.
Sample Code
The SDK includes sample projects demonstrating various functionalities:
- Annotations: Create custom annotations.
- Forms: Manipulate PDF forms.
- Security: Implement custom security handlers.
Key Components
- APIs: Application Programming Interfaces for interacting with Acrobat.
- HFTs: Host Function Tables for accessing Acrobat’s internal functions.
- Callbacks: Functions that Acrobat calls in response to events.
Best Practices for Plug-in Development
- Error Handling: Always check the return values of API calls.
- Performance: Optimize code to avoid slowing down Acrobat.
- Security: Validate all inputs and handle data securely.
- Documentation: Comment your code and maintain documentation for future reference.
Deploying Your Plug-in
Packaging
- Windows: Distribute the
.api
file. - macOS: Distribute the
.acroplugin
package.
Installation Script
Consider creating an installer to significantly simplify and enhance the installation process for users, making it more efficient and user-friendly.
Version Compatibility
Ensure your plug-in is compatible with the versions of Acrobat your users have installed.
Conclusion
By adding a custom menu item to your Adobe Acrobat plug-in, you’ve taken a significant step toward creating more interactive and user-friendly extensions. This simple example can serve as a foundation for more complex functionalities, such as processing documents, integrating with external services, or enhancing the user interface.